Errors are part of the deal when dealing with programming and software development. A common error developers encounter is the error message Object reference not set to an instance of an object.
If you see it, it can be pretty hard to understand what went wrong, especially if you are new here, but it is a nice example to practice, so let me explain it with the help of some examples.
So, what does this error mean, why does it happen and how can you resolve it? You will know how to deal with it and prevent it after understanding the root cause.
What Does the Error Mean?
To make sense of the error, it helps to dig into it. An “object” in programming is like a structure that describes something a person, a car, a file, and so on. These objects are constructed from “classes,” which are blueprints.
Before an object can be applied in a program, it needs to be developed, or “instantiated.” When you get the message “Object reference not set to an instance of an object,” it means that you’re trying to use an object that hasn’t been properly instantiated yet. It’s as if you’re trying to drive a car that has not been constructed yet.”
Why Does This Error Happen?
This error is common when you have a reference to an object in your code, but it isn’t pointing to anything valid. Some common causes include:
- Make sure to create an instance of the object before using it.
- Dereferencing an uninitialized pointer.
- This is like setting the value of an object to null (which is “nothing”).
- Handling a null from a method/function where an object was expected.
To start solving the issue, first understand, why the error happened.
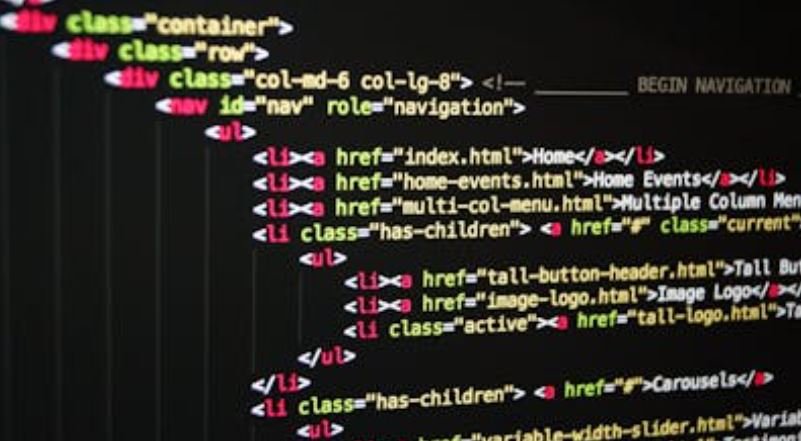
How the Error Might Look in Code
To illustrate this error more concretely let’s take an example. Let’s say you are writing a program in C# and you have a class named Car. The code snippet that tries to use the Car object looks something like this:
Car myCar; Console.WriteLine(myCar.Make);
As a result, running this code should trigger the error Object reference not set to an instance of an object. This is because we declared the myCar object, but we never instantiated it. The program is attempting to read the Make property of a Car object that hasn’t yet been instantiated.
How to Fix the Error
To resolve the “Object reference not set to an instance of an object” error, you need to make sure that you are creating the object before you use it or initializing it properly beforehand. Here’s what you can do about it:
Initialize the Object
A common answer to this is to instantiate the object before using it. So in the context of the Car example, this is what the corrected code would be:
Car myCar = new Car(); Console.WriteLine(myCar. Make);
The program knows what myCar is now because I am instantiating a new Car object.
Check for Null Values
If there’s a chance that an object can be null, you need to be sure that you check for this before attempting to use it. For example:
if (myCar! = null)
{
Console.WriteLine(myCar. Make);
} else
{
Console. Console.WriteLine("The car object is null." );
}
This way, the program never tries to fetch a null object and does not raise an error at all.
Debugging to Find the Problem
But sometimes it is not clear right away where the error is happening in your code. This makes debugging tools useful in finding what the problem is.
One way to control your execution is through a debugger, which allows you to execute your program one line at a time and inspect variable values at any line.
You can check the value of the object reference at the time the error is thrown and determine if it is null and if so why.
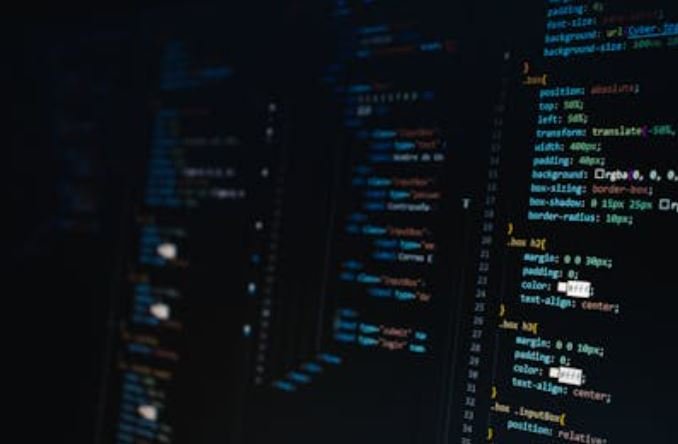
Preventing the Error
The best way to handle errors is to avoid them. Here are a few methods to help minimize the chances of seeing the “Object reference not set to an instance of an object” error:
Use Default Values
Try to use the default value assigned to objects while declaring it. For example, instead of this:
Car myCar;
Use this:
Car myCar = new Car();
That is,— making sure that the object is initialized when declared.
Null Merge Operators
Operating at the language level, many programming languages like C# and Python provide you with operators that allow you to work with nulls without fear. In C#, for example, it is the null-merge operator (??):
Console.WriteLine(myCar?.Make ?? "Default Make");
If myCar is not null, this statement will print the Make property value, otherwise “Default Make.”
Try-Catch Blocks for Error Handling
If you are dealing with objects that may be null, it is a good practice to wrap your code in a try-catch block to handle exceptions gracefully:
try {
Console.WriteLine(myCar. catch (NullReferenceException)
{
Console.WriteLine("The car object is null. ); }
This does not allow the program to crash, in case a null reference is encountered.
Benefits of Fixing This Error
There are many reasons why fixing the “Object reference not set to an instance of an object” error is important.
- Healthier Program Stability: Avoiding null references relates to not crashing/having surprises in your program.
- Improved User Experience: Users of your software will find no frustrating errors.
- Easier Debugging: Clean code, without errors, is easier to maintain and troubleshoot.
- Professionalism: An error-free handling lets your work appear professional and enhances its attractiveness.
Common Scenarios Where the Error Occurs
Now this error message can pop up in a myriad of circumstances, including:
- Lists — Not initializing a list before appending elements to it.
- Accessing Configuration Settings: Trying to use a configuration value without checking that it exists.
- External APIs: If you are getting null responses from the external APIs and you are not handling those responses properly.
- Working with Databases: Querying a database and expecting a result to be returned.
Knowing these scenarios helps you beat errors before they happen.
Conclusion
The “Object reference not set to an instance of an object” provides yet another obstacle for programmers, but one that becomes easier to comprehend as it is given time.
With these Error Handling Lessons, you can confidently handle this error by initializing objects adequately, checking for null values, and utilizing debugging tools.
Mistakes like that are part of your journey; every time you notice and rectify them, it gives you practical experience.
When proper coding and precaution measures are used, you can ensure that you create strong and reliable programs that provide smooth performance even in difficult situations.