Most of the languages include specific signs or templates to execute the tasks. It allows the developers to focus on what is necessary in a particular situation which results in a clean and efficient code.
Such a pattern ([_) may look quite confusing at first sight, however, there is no need for panic; knowing what it stands for helps you to get used to it.
We see the pattern ([_ in all functional and pattern-matching ish languages like Erlang and Python. This article describes what ([_) is, how it works, and when you might see it or want to use it.
Breaking Down ([_)
To understand ([_), let’s draw it out piece by piece:
1. [ and ]: These brackets are often used in a list. Lists are an ordered collection of values For example:
code[1, 2, 3]
This is a list containing three numbers: 1, 2, and 3.
2. _: The underscore acts as a wildcard. It matches any value and does not bind it to a variable. Acts as a placeholder that says: “I don’t care about this value.
3. ( and ): These parentheses are usually around the whole pattern or a tuple. In some languages, you can group patterns or data structures.
Together, ([_) means a list with at least one element (but the first one is always ignored). Any other items (if possible) are not referenced in this pattern.
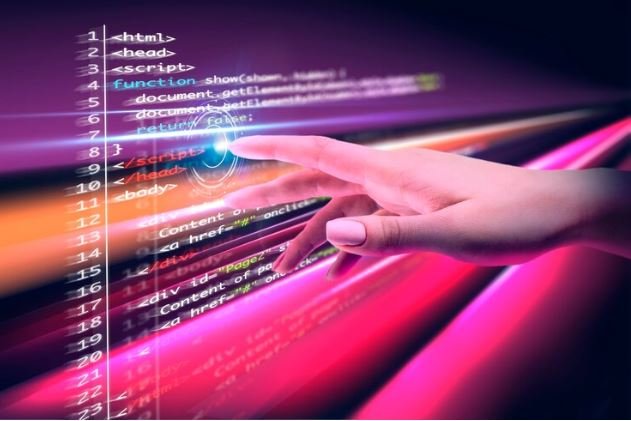
What Does ([_)
Represent?
In most contexts, ([_) means:
- Log in to access the content.
- The first part of the list is matched and then skipped with (_.
- The use of a pipe indicates the use of an alternator, the rest of the list is not mentioned in the pattern.
For example:
- [42] fits the pattern ([_), since it’s a list with one element, and _ discards the value 42.
- [10, 20, 30] also matches, because it’s a list, and _ will ignore the first item, which is 10.
- But an empty list ([]) does not fit this pattern, as there is no first element for _ to ignore.
List of examples in multiple languages
We can look at what (_[) may look like and behave like in a few popular programming languages.
Erlang
Pattern matching is a major feature of Erlang and is used in functions, case statements, and list operations. This pattern ([_) matches a list that has at least one element.
Example:
match_list([_ | _]) ->
"The list has at least one element";
match_list([]) ->
"The list is empty".
Here:
- The first clause matches any list with at least one element, ignoring the first.
- The second clause is a match for an empty list.
Calling the function:
match_list([42, 50]). % Output: "The list has at least one element"
match_list([]). % Output: "The list is empty"
Python
In Python, _ is heavily used as a placeholder, a thing that signifies that something does not matter. Unlike pattern matching in languages like Scala, Python doesn’t offer direct pattern matching on lists, but you can achieve a similar effect using unpacking.
Example:
def match_list(lst):
if lst:
_ = lst[0] # Ignore the first element
return "The list has at least one element"
else:
return "The list is empty"
print(match_list([10, 20, 30])) # Output: "The list has at least one element"
print(match_list([])) # Output: "The list is empty"
Here, _
is used to explicitly show that the first element is not important.
Haskell
For example, in Haskell, we usually use the pattern match to consume the list. The pattern ([_ would match something like:
matchList :: [a] -> String
matchList (_:_) = "The list has at least one element"
matchList [] = "The list is empty"
Here:
- (:) Only matches a list of one or more elements, discarding the first.
- [] matches an empty list.
Calling the function:
matchList [1, 2, 3] -- Output: "The list has at least one element"
matchList [] -- Output: "The list is empty"
Practical Uses of ([_)
However, the pattern ([_ is common with lists. Governments and companies are already using these:
Filtering Non-Empty Lists
For example, you`ve got a list of lists and you don’t want the empty ones. It appears to be a hint that the pattern ([_) can be used to test for non-empty lists.
Example in Erlang:
filter_non_empty(Lists) ->
[List || List = [_ | _] <- Lists].
Input:
Lists = [[], [1, 2], [3], []].
Output:
code[[1, 2], [3]]
Defining Functions with Specific Input
You can write functions that only iterate on non-empty lists, skipping the head.
Example in Python:
def process_list(lst):
if lst:
_ = lst[0] # Ignore the first element
return "Processing the list"
else:
return "Cannot process an empty list"
print(process_list([5, 10, 15])) # Output: "Processing the list"
print(process_list([])) # Output: "Cannot process an empty list"
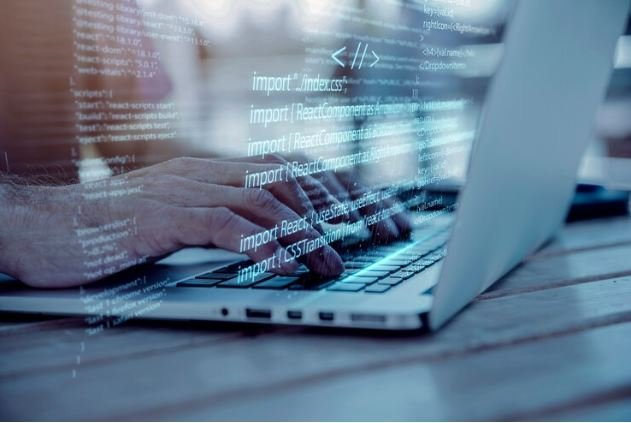
Pattern Matching for Complex Structures
Continuations ([_) are used in functional programming as a part of a more complex pattern. For instance, matching tuples whose elements with non-empty lists:
match_tuple({_, [_ | _]}) ->
"The tuple contains a non-empty list";
match_tuple(_) ->
"The tuple does not match".
Input:
match_tuple({ok, [1, 2, 3]}). % Output: "The tuple contains a non-empty list"
match_tuple({error, []}). % Output: "The tuple does not match"
Common Mistakes to Avoid
When using ([_), try not to fall into these common traps:
- Empty Lists: Keep in mind that (_ xs) does not match an empty list. If necessary, as in the case where [] is a valid element name, always provide a separate pattern for [].
- Syntax Error: Make sure the pattern is written properly according to the language you are dealing with.
- Too much: Use: only if the ignored value doesn’t matter. Using _ too generously makes code less readable.
Best Practices for Using Patterns Like ([_)
- By using a style such as ([_ — to simplify logic, which in turn makes the code easier to read.
- Use other patterns to ensure strong error handling.
- Make sure to test your patterns against various inputs so you know what to expect.
Conclusion
The combination of pattern ([) is a powerful and essential tool in all programming methods and languages that contain matching. It enables you to manipulate lists elegantly and expressively. Once you comprehend how ([) functions and in what context to apply it, the code that you produce can become cleaner and more performant as a consequence.
This is how the concepts of ([_ come in handy for manipulating lists and data of all kinds, whether you filtering lists, defining functions on them, or matching more complex structures. You will find this pattern is a staple in your coding toolbelt with practice.